Masonry with Lazyloaded Images
Demo link: https://jsbin.com/viderej/edit?html,output
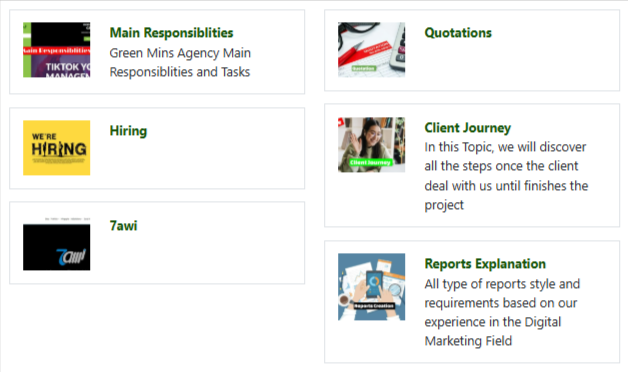
<div class="row masonry-grid" data-masonry='{"percentPosition": true }'>
<?php query_posts('cat=10&showposts=-1'); ?>
<?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?>
<div class="col-lg-6 col-sm-12 grid-item">
<div class="row mx-0 mb-3 p-3 border ">
<div class="col-3 p-0">
<?php
$image_data = wp_get_attachment_image_src(get_post_thumbnail_id($post->ID), "thumbnail");
if ($image_data):
$image_url = $image_data[0];
$image_width = $image_data[1];
$image_height = $image_data[2];
// Generate SVG placeholder with dynamic viewBox
$svg_placeholder = 'data:image/svg+xml;base64,' . base64_encode(
'<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 ' . $image_width . ' ' . $image_height . '" preserveAspectRatio="xMidYMid slice">
<rect width="100%" height="100%" fill="#f8f9fb"/>
</svg>'
);
?>
<a href="<?php echo get_permalink($post->ID); ?>">
<img
src="<?php echo $svg_placeholder; ?>"
data-src="<?php echo $image_url; ?>"
class="lazyload img-fluid"
alt="<?php the_title(); ?>">
</a>
<?php endif; ?>
</div>
<div class="col-9 ps-4"><a class="fw-bold" href="<?php echo get_permalink($post->ID) // get the loop-item the link ?>"><?php the_title(); ?></a>
<p class="m-0"><?php the_field('description'); ?></p>
</div>
</div>
</div>
<?php endwhile; endif; ?>
<?php wp_reset_query(); ?>
</div>
Lazy-Loading Featured Image with SVG Placeholder (Preserving Aspect Ratio)
This code snippet retrieves a WordPress post’s featured image data and uses it to generate an inline (base64-encoded) SVG placeholder. By setting the real image’s src to the SVG placeholder and the actual URL to data-src, lazyloading scripts can swap in the real image once it’s needed. The addition of width and height attributes on the tag preserves the correct aspect ratio before the real image loads, avoiding layout shifts. The img-fluid class ensures the image remains responsive within Bootstrap layouts.
<div class="col-3 p-0">
<?php
$image_data = wp_get_attachment_image_src(get_post_thumbnail_id($post->ID), "thumbnail");
if ($image_data):
$image_url = $image_data[0];
$image_width = $image_data[1];
$image_height = $image_data[2];
// Generate SVG placeholder with dynamic viewBox
$svg_placeholder = 'data:image/svg+xml;base64,' . base64_encode(
'<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 ' . $image_width . ' ' . $image_height . '" preserveAspectRatio="xMidYMid slice">
<rect width="100%" height="100%" fill="#f8f9fb"/>
</svg>'
);
?>
<a href="<?php echo get_permalink($post->ID); ?>">
<img
src="<?php echo $svg_placeholder; ?>"
data-src="<?php echo $image_url; ?>"
width="<?php echo $image_width; ?>"
height="<?php echo $image_height; ?>"
class="lazyload img-fluid"
alt="<?php the_title(); ?>">
</a>
<?php endif; ?>
</div>